/**
* Java class example
* The class illustrates how to write comments used
* to generate JavaDoc documentation
*
* @author Catalin
* @version 2.00, 23 Dec 2010
*/
public class MyClass {
/**
*
* Simple method.
*
* The method prints a received message on the Console
*
* @param message String variable to be printed
* @see MyClass
* @deprecated
* @since version 1.00
*/
public void MyMethod(String message)
{
System.out.printf(message);
}
/**
*
* Simple method example.
* The method prints a received message on the Console
*
* @param message String variable to be printed
* @since version 1.00
*/
public void printMessage(String message)
{
System.out.printf(message);
}
/**
*
* Simple method example.
*
* The methods adds 2 numbers and return the result
*
* @param val1 the first value
* @param val2 the second value
* @return sum between val1 and val2
* @since version 2.00
*/
public int add(int val1, int val2)
{
return val1+val2;
}
}
How to generate JavaDoc in Eclipse Helios
1. Open the Eclipse project
2. Select
Project –> Generate JavaDoc
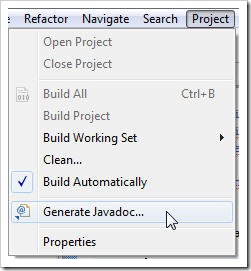
Generate JavaDoc in Eclipse
3. At first step of the wizard, you can define settings for:
3.1 path for the
javadoc.exe tool from the JDK;
3.2 project resources for which to generate JavaDoc;
3.3 classes and methods for which to generate JavaDoc based on their visibility;
3.4 location of the JavaDoc (by default it will be placed in the
doc folder in the project location)

Generate JavaDoc in Eclipse - Step 1
4. At second step you can make settings regarding:
4.1 documentation structure;
4.2 JavaDoc tags to be processed;
4.3 other resources(archives, projects) used in project to be included in the documentation;
4.4 another CSS style sheet for the documentation;

Generate JavaDoc in Eclipse - Step 2
5. At the last step you can save the settings in an Ant script for future use.

Generate JavaDoc in Eclipse - Step 3
No comments:
Post a Comment