In this section we will develop a simple Hello World Web service and then deploy on the Axis2 engine.
Apache Axis2 Hello World Example
Apache Axis2 Hello World ExampleIn this section we will develop a simple Hello World Web service and then deploy on the Axis2 engine. In the last section we have deployed the Axis2 engine on the Tomcat server. We will use the same Axis2 engine and then deploy and test the application.
About the Hello World Web service
Our Web service example is very simple example that will explain you the process of development and deployment of Web services on the Axis2 engine. The Hello World Web service will just return the "Hello World" message to the Web service client.
Directory Structure of the application
Create the following directory structure in your hard disk. You can also download the source code of the example from here if you don't want to do it manually. The source code download contains all files in proper directories.

Developing Web service
The development of Web services is easy process. You can start from the WSDL file(Contract first approach) or from the Service code(Code first approach). Most developer prefers the code first approach. We will start with the source and create the Web service. Here are the steps involved in creating the new Web services with code first approach in Apache Axis2:
- Develop the Service Class
- Develop the service descriptor e.g. services.xml
- Compile and Service class and create the Web services achieve file (.aar)
Our services class is "HelloWorldService.java", let's create the same.
Create a java file HelloWorldService.java into \HelloWorld\webservice\net\roseindia directory and add the code shown below.
Here is the code of Hello World Webservice.
package net.roseindia; |
In Axis2 the service configuration file used is services.xml. The services.xml file must present in the META-INF directory of the service achieve. You can have multiple services in the services.xml file. The <service>...</service> tag is used to configure the Web service.
<service >
<!-- Configuration of the service -->
</service>
Here is the services.xml file for our application:
<service>
<parameter name="ServiceClass" locked="false">net.roseindia.HelloWorldService</parameter>
<operation name="sayHello">
<messageReceiver class="org.apache.axis2.rpc.receivers.RPCMessageReceiver"/>
</operation>
</service>
Our service class is net.roseindia.HelloWorldService and the message receiver is org.apache.axis2.rpc.receivers.RPCMessageReceiver. The operation we are exposing is sayHello.
Compiling and building the service archieve
Go to the E:\Axis2Tutorial\Examples\HelloWorld\webservice directory and compile the java class using following command:
E:\Axis2Tutorial\Examples\HelloWorld\webservice>javac net/roseindia/*.java
Create the service achieve using the following command:
E:\Axis2Tutorial\Examples\HelloWorld\webservice>jar cvf HelloWorldService.aar *
Here is the details:
E:\Axis2Tutorial\Examples\HelloWorld\webservice>javac net/roseindia/*.java
E:\Axis2Tutorial\Examples\HelloWorld\webservice>jar cvf HelloWorldService.aar *
added manifest
ignoring entry META-INF/
adding: META-INF/services.xml(in = 242) (out= 162)(deflated 33%)
adding: net/(in = 0) (out= 0)(stored 0%)
adding: net/roseindia/(in = 0) (out= 0)(stored 0%)
adding: net/roseindia/HelloWorldService.class(in = 489) (out= 321)(deflated 34%)
adding: net/roseindia/HelloWorldService.java(in = 167) (out= 126)(deflated 24%)
E:\Axis2Tutorial\Examples\HelloWorld\webservice>
Deploying the Web Services
Now copy the HelloWorldService.aar into webapps/axis2/WEB-INF/services directory and restart the Tomcat. The Apache Axis2 engine will deploy the service on the server. Now open the browser and browser the url http://localhost:8080/axis2/services/listServices. Your browser should display the service name as shown below:
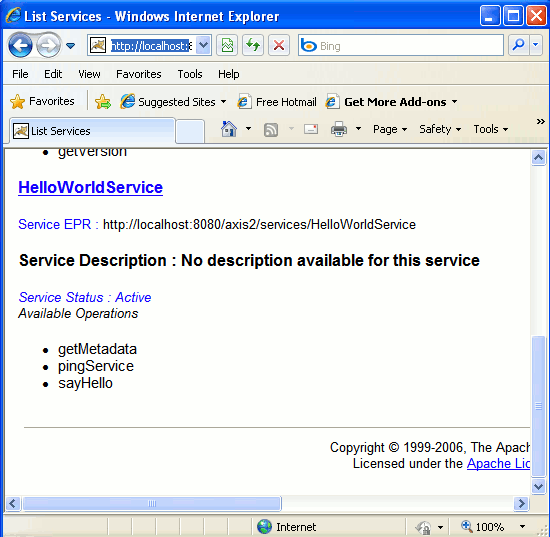
You can view the WSDL file at http://localhost:8080/axis2/services/HelloWorldService?wsdl.
n this section we will develop client code example to access the Hello World Web service developed in the last section.
Axis2 client - Axis2 Client example
Apache Axis2 Client codeIn this section we will develop client code example to access the Hello World Web service developed in the last section. In the last section we developed and deployed the Hello World Web service. In this section we will write the Web service client code and call the web service.
Setting up the environment
In our first section of downloading and installing Axis 2 engine, we instructed you to download the binary version of Apache Axis2. Now extract the binary version(axis2-1.5.1-bin.zip) using any zip tool. After extracting the file into E:\Axis2Tutorial (assuming your tutorial directory is E:\Axis2Tutorial), you will get a directory "E:\Axis2Tutorial\axis2-1.5.1-bin\axis2-1.5.1" which contains the binary version of the Apache Axis2 engine. Now set the following environment variables:
a) AXIS2_HOME=E:\Axis2Tutorial\axis2-1.5.1-bin\axis2-1.5.1
b) Add E:\Axis2Tutorial\axis2-1.5.1-bin\axis2-1.5.1\bin into path
c) Add E:\Axis2Tutorial\axis2-1.5.1-bin\axis2-1.5.1\lib\* into CLASS_PATH
After making the above changes the wsdl2java.bat is available for generating the client code calling Web service.
Generating the client code using wsdl2java.bat tool
Now create a new directory E:\Axis2Tutorial\Examples\HelloWorld\client and then open dos prompt and go to same directory. Now generate the client code using following command:
WSDL2Java.bat -uri http://localhost:8080/axis2/services/HelloWorldService?wsdl -o client
Here is the output of above command.
E:\>cd E:\Axis2Tutorial\Examples\HelloWorld\client
E:\Axis2Tutorial\Examples\HelloWorld\client>WSDL2Java.bat -uri http://localhost:
8080/axis2/services/HelloWorldService?wsdl -o client
Using AXIS2_HOME: E:\Axis2Tutorial\axis2-1.5.1-bin\axis2-1.5.1
Using JAVA_HOME: E:\JDK\jdk1.6.0_03
Retrieving document at 'http://localhost:8080/axis2/services/HelloWorldService?wsdl'.
The above command will generate a) HelloWorldServiceStub.java and b) HelloWorldServiceCallbackHandler.java into E:\Axis2Tutorial\Examples\HelloWorld\client\client\src\net\roseindia directory.
Now run cd to client/src directory.
cd client/src
Developing the code to call the service
Create the file Test.java into E:\Axis2Tutorial\Examples\HelloWorld\client\client\src\net\roseindia directory. The code of Test.java file is as below:
package net.roseindia; |
Now go to E:\Axis2Tutorial\Examples\HelloWorld\client\client\src directory and with the help of javac command compile the code.
E:\Axis2Tutorial\Examples\HelloWorld\client\client\src>javac net/roseindia/*.java
Note: net\roseindia\HelloWorldServiceStub.java uses unchecked or unsafe operations.
Note: Recompile with -Xlint:unchecked for details.
To run the client type following command:
java net/roseindia/Test
Here is the output:
E:\Axis2Tutorial\Examples\HelloWorld\client\client\src>javac -extdirs E:\applications\axis2\axis2-1.5.2-bin\axis2-1.5.2\lib net/roseindia/*.java
Note: net\roseindia\HelloWorldServiceStub.java uses unchecked or unsafe operations.
Note: Recompile with -Xlint:unchecked for details.
E:\Axis2Tutorial\Examples\HelloWorld\client\client\src>java -Djava.ext.dirs=E:\applications\axis2\axis2-1.5.2-bin\axis2-1.5.2\lib net/roseindia/Test
log4j:WARN No appenders could be found for logger (org.apache.axis2.description.AxisService).
log4j:WARN Please initialize the log4j system properly.
Response : Hello : Deepak Kumar
E:\Axis2Tutorial\Examples\HelloWorld\client\client\src>
The client appliction makes a call to Web service and in response Web services returns the Hello : Deepak Kumar message
You have successfully tested your Web Service.
No comments:
Post a Comment